0x00.相关
- 列表(Listview)
- 模板(DataTemplate)
- 模板选择器(DataTemplateSelector)
0x01.声明类
class Html_TimeLineClass { public string Content { get; set; } public string DateTime { get; set; } public string Id { get; set; } public int Index { get; set; } }
0x02.自定义模板
<DataTemplate x:Key="RightTimeLineItemTemplate"> <ListViewItem> <Grid> <Grid.ColumnDefinitions> <ColumnDefinition Width="1*"/> <ColumnDefinition Width="14"/> <ColumnDefinition Width="1*"/> </Grid.ColumnDefinitions > <Border Height="14" Width="14" CornerRadius="6" BorderThickness="2" BorderBrush="LightGray" Grid.Column="1" VerticalAlignment="Bottom"> <Border.Background> <AcrylicBrush BackgroundSource="HostBackdrop" TintColor="{ThemeResource SystemChromeMediumColor}" TintOpacity=".7"/> </Border.Background > </Border > <StackPanel Orientation="Horizontal" HorizontalAlignment="Left" Grid.Column="2"> <StackPanel Orientation="Vertical"> <StackPanel Orientation="Horizontal"> <HyperlinkButton Content="{Binding Id}"/> <TextBlock Text="{Binding DateTime}" Margin="8,0,0,0" Foreground="Gray" VerticalAlignment="Center"/> </StackPanel > <TextBlock Text="{Binding Content}"/> <Border Height="1" Background="LightGray" CornerRadius="2"/> </StackPanel > </StackPanel> </Grid > </ListViewItem > </DataTemplate > <DataTemplate x:Key="LeftTimeLineItemTemplate"> <ListViewItem> <Grid> <Grid.ColumnDefinitions> <ColumnDefinition Width="1*"/> <ColumnDefinition Width="14"/> <ColumnDefinition Width="1*"/> </Grid.ColumnDefinitions > <Border Height="14" Width="14" CornerRadius="6" BorderThickness="2" BorderBrush="LightGray" Grid.Column="1" VerticalAlignment="Bottom"> <Border.Background> <AcrylicBrush BackgroundSource="HostBackdrop" TintColor="{ThemeResource SystemChromeMediumColor}" TintOpacity=".7"/> </Border.Background > </Border > <StackPanel Orientation="Horizontal" HorizontalAlignment="Right" Grid.Column="0" > <StackPanel Orientation="Vertical"> <StackPanel Orientation="Horizontal"> <HyperlinkButton Content="{Binding Id}"/> <TextBlock Text="{Binding DateTime}" Margin="8,0,0,0" Foreground="Gray" VerticalAlignment="Center"/> </StackPanel > <TextBlock Text="{Binding Content}"/> <Border Height="1" Background="LightGray" CornerRadius="2"/> </StackPanel > </StackPanel> </Grid > </ListViewItem > </DataTemplate >
0x03.自定义模板选择器
XAML
<local:TimeLineItemTemplateSelector x:Key="TimeLineItemTemplateSelector" LeftTimeLineItemTemplate="{StaticResource LeftTimeLineItemTemplate}" RightTimeLineItemTemplate="{StaticResource RightTimeLineItemTemplate}" />
C#
public class TimeLineItemTemplateSelector : DataTemplateSelector { public DataTemplate LeftTimeLineItemTemplate { get; set; } public DataTemplate RightTimeLineItemTemplate { get; set; } protected override DataTemplate SelectTemplateCore(object item) { int index= ((Html_TimeLineClass)item).Index; if (index % 2 == 1) return RightTimeLineItemTemplate; else return LeftTimeLineItemTemplate; }//奇按照偶性左右分布 }
0x04.绘制中轴和Listview容器
<Grid> <Border HorizontalAlignment="Center" Width="3" Background="Gray" CornerRadius="2" Margin="0,10,0,10"/> <ListView x:Name="TimeLineListview" Margin="0,10,0,10" SelectionMode="None" ItemContainerStyle="{StaticResource LVCstretch}" ItemTemplateSelector="{StaticResource TimeLineItemTemplateSelector}" IsItemClickEnabled="True"> </ListView > </Grid >
0x05.数据绑定
TimeLineListview.ItemsSource = your datasource;
0x06.效果图
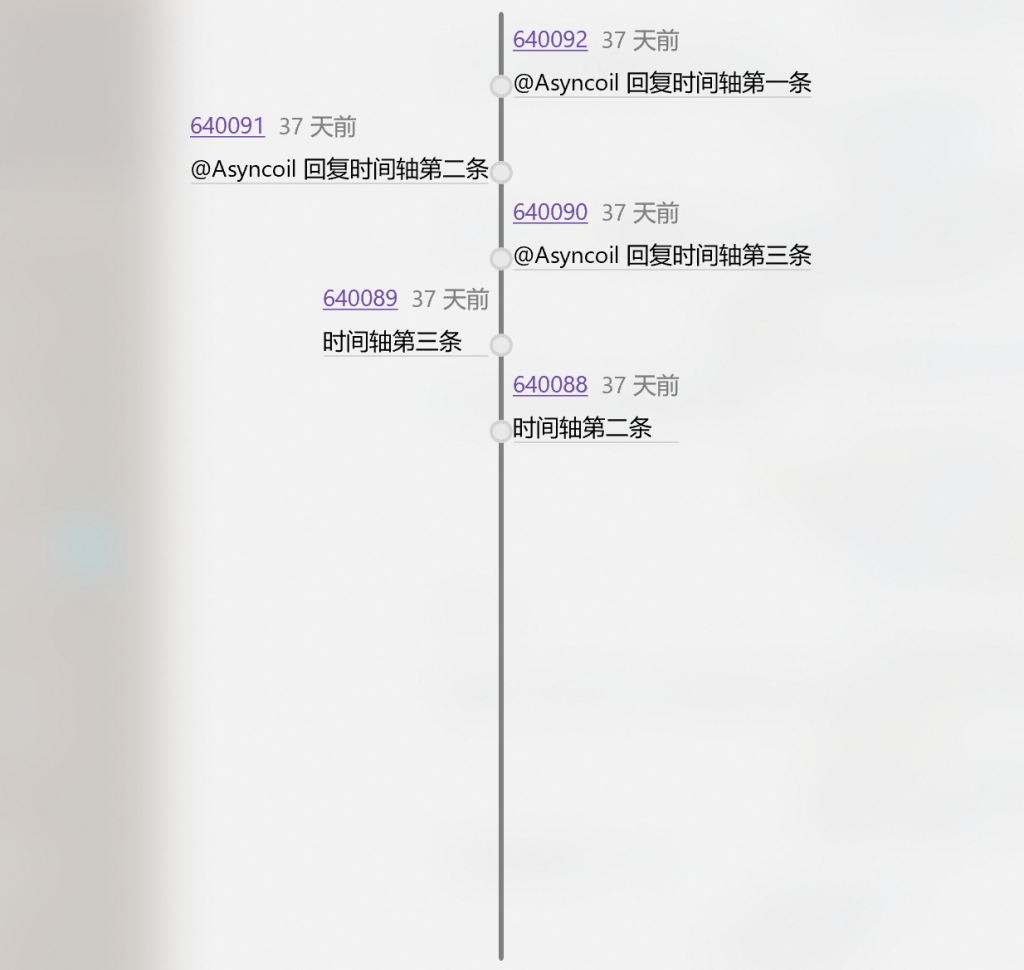